1. Container란?
Container Widget은 1개의 하위 Widget을 생성할 수 있는 Flutter의 기본 Widget입니다. 테두리, 여백, 정렬, 크기 등의 다양한 요소를 설정하여 하위 Widget을 꾸밀 수 있습니다.
하위 Widget이 있는 경우, 하위 Widget에 맞는 크기로 설정됩니다.
하위 Widget이 없는 경우, 최대치로 차지 가능한 범위의 크기로 설정됩니다.
2. Container 생성자 및 속성
https://api.flutter.dev/flutter/widgets/Container-class.html
Container class - widgets library - Dart API
A convenience widget that combines common painting, positioning, and sizing widgets. A container first surrounds the child with padding (inflated by any borders present in the decoration) and then applies additional constraints to the padded extent (incorp
api.flutter.dev
Flutter Material API 가이드를 통해 Container Widget의 생성자 및 중요 속성에 대해 정리해보도록 하겠습니다.
○ 생성자(Constructor)
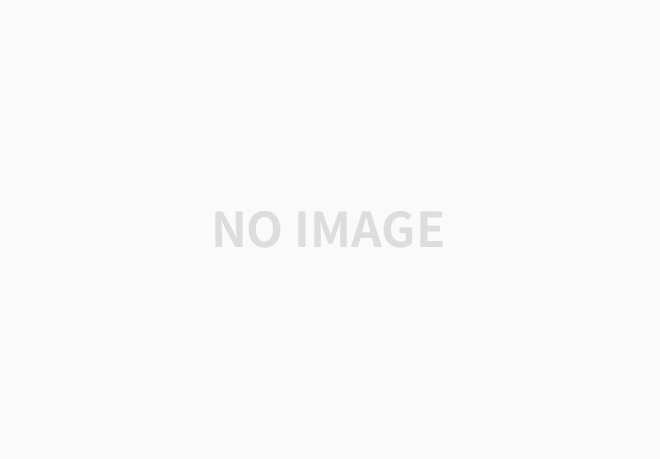
○ 속성(Property)
1) alignment
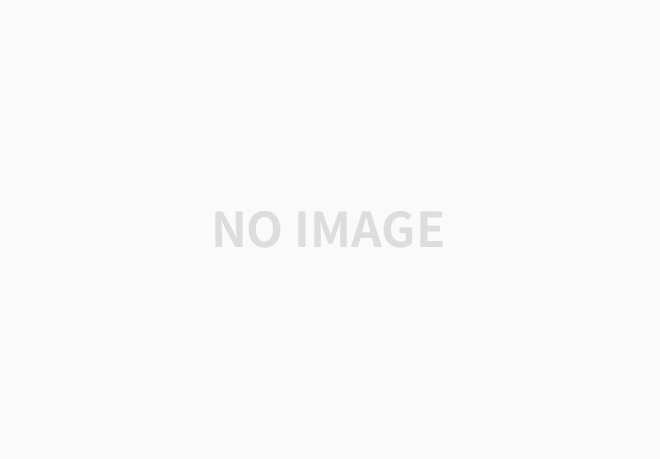
- 매개변수 타입 : AlignmentGeometry
- 역할 : Container Widget 내 하위 Widget 위치 지정
- 정중앙 : Alignment.center 또는 Alignment(0.0, 0.0)
- 하단중앙 : Alignment.bottomCenter 또는 Alignment(0.0, 1.0)
- 상단중앙 : Alignment.topCenter 또는 Alignment(0.0, -1.0)
- 왼쪽 끝 : Alignment.centerLeft 또는 Alignment(-1.0, 0.0)
- 오른쪽 끝 : Alignment.centerRight 또는 Alignment(1.0, 0.0)
- 왼쪽 하단 : Alignment.bottomLeft 또는 Alignment(-1.0, 1.0)
- 왼쪽 상단 : Alignment.topLeft 또는 Alignment(-1.0, -1.0)
- 오른쪽 하단 : Alignment.bottomRight 또는 Alignment(1.0, 1.0)
- 오른쪽 상단 : Alignment.topRight 또는 Alignment(1.0, -1.0)
2) child
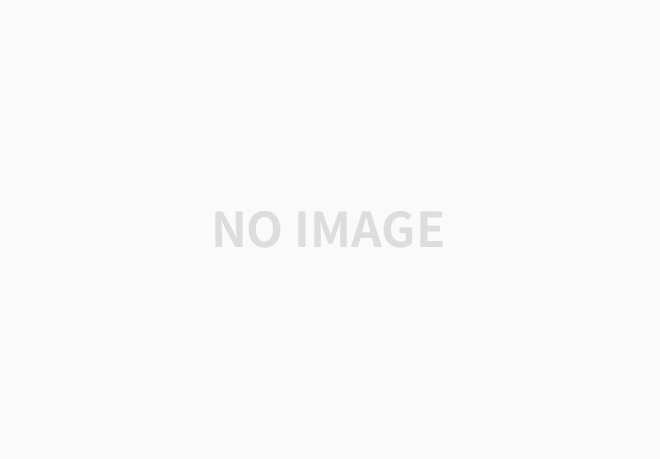
- 매개변수 타입 : Widget
- 역할 : Container Widget 내 1개의 하위 Widget 컨텐츠 추가 가능
3) color
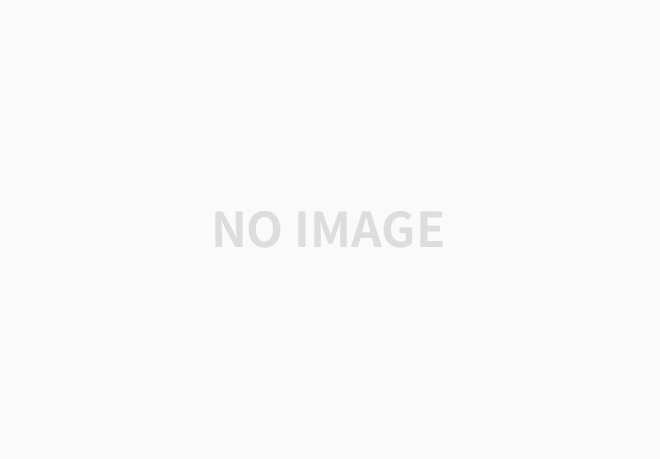
- 매개변수 타입 : Color
- 역할 : 하위 Widget의 배경색 설정
4) constraints
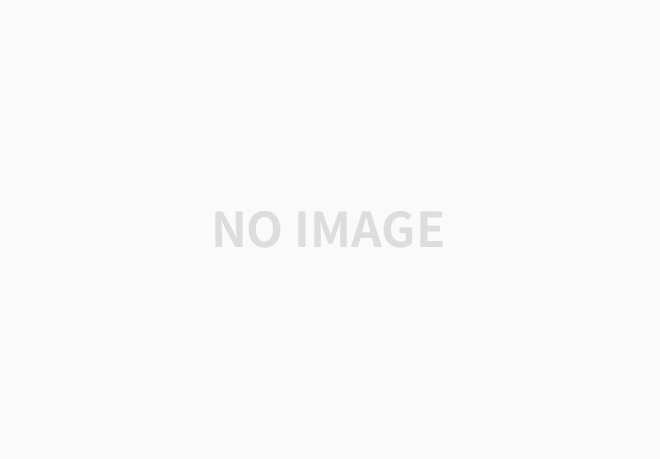
- 매개변수 타입 : BoxConstraints
- 역할 : 하위 Widget에 대한 추가 제약 설정
5) decoration
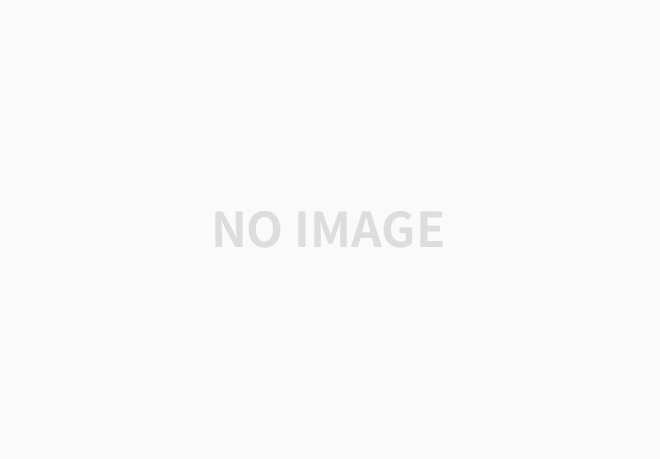
- 매개변수 타입 : Decoration
- 역할 : 하위 Widget에 대한 꾸밈 설정
* decoration 속성의 색상 설정이 color 속성의 색상 설정보다 우선 적용
6) margin

- 매개변수 타입 : EdgeInsetxGeometry
- 역할 : Container Widget 기준 외부 여백
- EdgeInsets.all(double value) -> 예) EdgeInsets.all(8.0)
- EdgeInsets.symmetric({double vertical = 0.0, double horizontal = 0.0}) -> 예) EdgetInsets.symmetric(vertical: 8.0, horizontal: 4.0)
- EdgeInsets.only({double left = 0.0, double top = 0.0, double right = 0.0, double bottom = 0.0}) -> 예) EdgeInsets.only(left: 40.0, top: 30.0, right: 20, bottom: 10)
7) padding

- 매개변수 타입 : EdgeInsetsGeometry
- 역할 : Container Widget과 하위 Widget 간의 여백
3. Container 예제 코드
import 'package:flutter/material.dart';
void main() {
runApp(
MaterialApp(
title: 'Description',
home: Scaffold(
backgroundColor: Colors.grey,
appBar: AppBar(
backgroundColor: Colors.teal,
title: const Text(
'TITLE',
),
),
body: SafeArea(
child: Container(
alignment: Alignment.topLeft,
// color: Colors.blue,
height: 300.0,
width: 300.0,
constraints: const BoxConstraints(
maxHeight: 200.0,
maxWidth: 200.0,
minHeight: 100.0,
minWidth: 100.0,
),
decoration: BoxDecoration(
color: Colors.green,
image: const DecorationImage(
image: NetworkImage('https://picsum.photos/200'),
),
border: Border.all(
color: Colors.black,
style: BorderStyle.solid,
width: 10.0,
),
shape: BoxShape.rectangle,
borderRadius: BorderRadius.circular(10.0),
),
child: const Center(
child: Text(
'Container',
style: TextStyle(
fontSize: 30.0,
color: Colors.black,
),
textAlign: TextAlign.center,
),
),
margin: const EdgeInsets.all(50.0),
padding: const EdgeInsets.all(20.0),
),
),
bottomNavigationBar: BottomNavigationBar(
items: const <BottomNavigationBarItem>[
BottomNavigationBarItem(
icon: Icon(Icons.home),
label: 'Home',
),
BottomNavigationBarItem(
icon: Icon(Icons.info),
label: 'Info',
),
BottomNavigationBarItem(
icon: Icon(Icons.settings),
label: 'Settings',
),
],
),
),
),
);
}
예제 코드의 의미는 다음과 같습니다.
- 'Description'이라는 문자열로 App 설명서 작성
- Scaffold Widget으로 메인 화면을 구현
- 배경 색상을 회색으로 설정
- AppBar Widget으로 상단 메뉴 구현
- 메뉴 색상을 암청색(teal)으로 설정
- Text Widget으로 'TITLE'이란 제목 표시
- SafeArea Widget으로 여백이 확보된 컨텐츠 영역 지정
- child 속성을 Container Widget으로 지정
- Container Widget의 위치를 SafeArea 내 좌상단으로 설정
- Container Widget의 배경 색상은 파란색으로 설정하였으나, 주석 처리
- 크기는 300 x 300으로 설정하였으나, 별도 설정한 제약 조건(가로/세로의 크기를 최소 100, 최대 200)으로 인해 최종 크기는 200 x 200으로 설정
- 꾸밈(Decoration) 설정
- Container Widget의 배경 색상을 초록색으로 설정
- Decoration에서 색상을 설정하면, Container Widget에서 색상을 설정한 부분과 충돌 발생(에러 발생으로 인해 한 가지로만 설정 필요 -> 파란색 주석 처리)
- 배경 이미지를 웹('https://picsum.photos/200')에서 불러오기(대신, 초록색 배경 색상이 가려짐)
- 두께가 10인, 실선 타입의 경계선 추가
- 모양은 사각형으로 설정
- 테두리 형태는 둥글게 설정
- child 속성을 Center Widget으로 지정(상하좌우 중앙 정렬 역할)
- child 속성을 Text Widget으로 지정
- 'Container'란 문자열 표시
- 문자열 크기 30, 검정 글씨, 가운데 정렬
- child 속성을 Text Widget으로 지정
- 외부 여백 크기를 50으로 설정
- 내부 여백 크기를 20으로 설정
- child 속성을 Container Widget으로 지정
- BottomNavigationBar로 하단 메뉴 구현
- BottomNavigationBar Widget의 items 속성 매개변수 타입은 List<BottomNavigationBarItem>
- BottomNavigationBarItem Widget은 아이콘 이미지와 라벨을 구현하는 역할
- Home 이미지와 'Home' 문자열 라벨 지정
- Info 이미지와 'Info' 문자열 라벨 지정
- Settings 이미지와 'Settings' 문자열 라벨 지정
- BottomNavigationBarItem Widget은 아이콘 이미지와 라벨을 구현하는 역할
- BottomNavigationBar Widget의 items 속성 매개변수 타입은 List<BottomNavigationBarItem>
4. Container 실행 결과


5. Scaffold, SafeArea, Container 비교
1) Scaffold
App의 상단 메뉴, 본문, 하단 메뉴 등 Material Design 기반의 전체적인 레이아웃 구조를 제공합니다.
2) SafeArea
다양한 모바일 기기 환경에 상관없이 컨텐츠가 깨지거나 가려지지 않게 여백이 확보된 영역을 의미합니다.
3) Container
1개의 하위 Widget을 가질 수 있는 기본 Widget입니다. 컨텐츠를 구성하는 기본 레이아웃 단위로 볼 수 있습니다.
'App Programming > Flutter' 카테고리의 다른 글
[Flutter] Widget - Row (0) | 2022.04.18 |
---|---|
[Flutter] Widget - Column (0) | 2022.04.18 |
[Flutter] Widget - SafeArea (0) | 2022.04.17 |
[Flutter] Widget - Scaffold (0) | 2022.04.17 |
[Flutter] Widget - MaterialApp (0) | 2022.04.06 |
댓글